Implement FCM push notification for iOS APP in Swift.
Add Firebase GoogleService-Info.plist to the project root
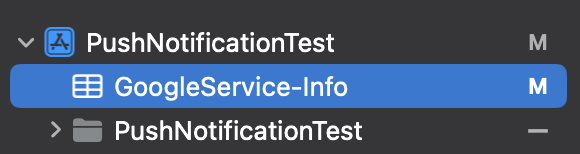
Add Firebase SDK
https://github.com/firebase/firebase-ios-sdk
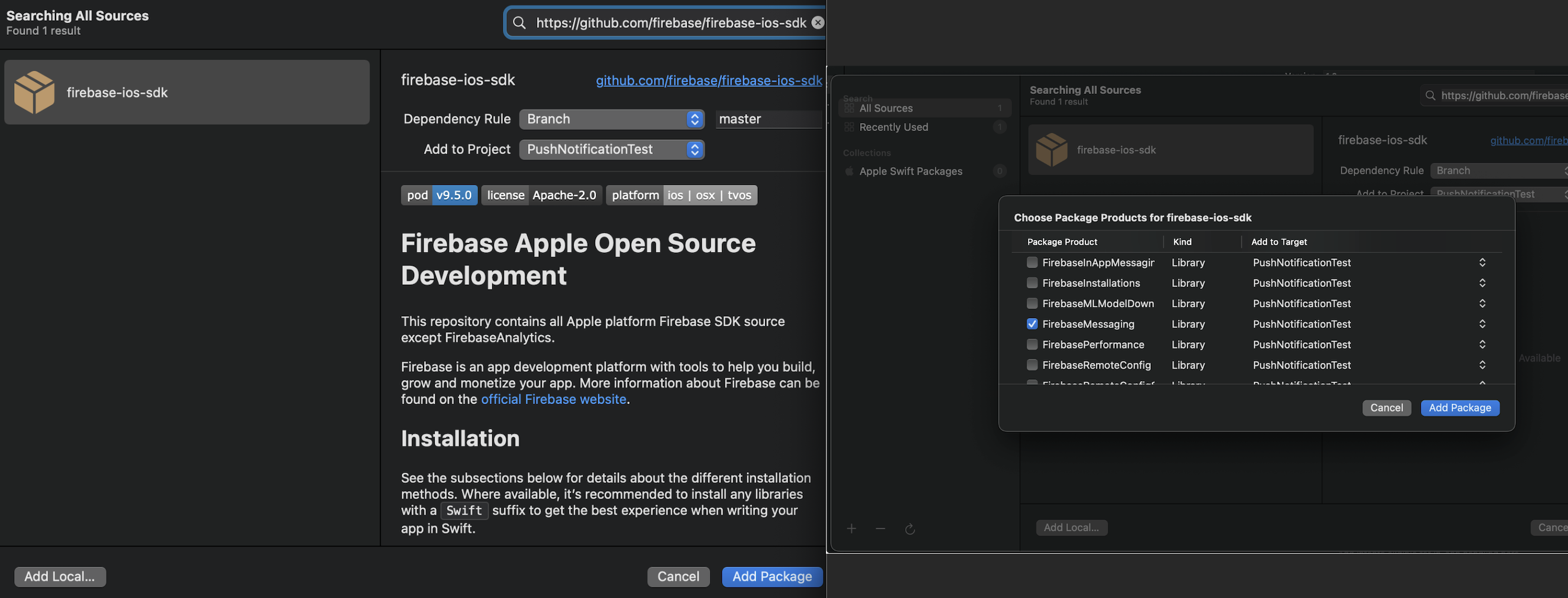
Initialize FCM & ask for notification permission
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure() UNUserNotificationCenter.current().getNotificationSettings(completionHandler: { settings in switch settings.authorizationStatus {
case .denied: print("denied") case .notDetermined: UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .sound, .badge], completionHandler: { granted, error in if granted { print("Request notification permission is allowed!") } else { print("Request notification permission is rejected!") } })
default: break } }) application.registerForRemoteNotifications() UNUserNotificationCenter.current().delegate = self Messaging.messaging().delegate = self return true }
|
Implement UNUserNotificationCenterDelegate
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| extension AppDelegate: UNUserNotificationCenterDelegate { func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) { completionHandler([.sound, .badge, .alert]) } func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { print("didReceive notification") let userInfo = response.notification.request.content.userInfo let id = response.notification.request.content.categoryIdentifier print("info \(userInfo) \(id)") ... completionHandler() } func application( _ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) { Messaging.messaging().apnsToken = deviceToken } }
|
Handle FCM token in MessagingDelegate
1 2 3 4 5 6 7 8
| extension AppDelegate: MessagingDelegate { func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String?) { if let token = fcmToken{ print(token) } } }
|