Implement FCM push notification for Android APP in Kotlin.
Add Firebase GoogleService-Info.plist to the project app folder
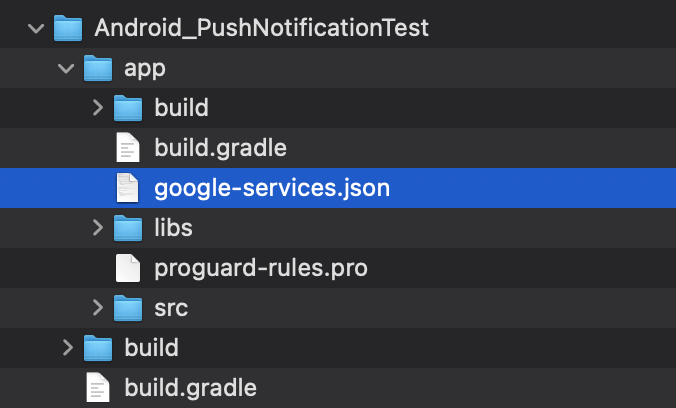
Add FCM to dependencies
1 2 3 4 5 6
| dependencies { ... implementation 'com.google.firebase:firebase-messaging-ktx:23.0.8' implementation platform('com.google.firebase:firebase-bom:30.4.1') implementation 'com.google.firebase:firebase-analytics-ktx' }
|
Add permission to Manifest
1
| <uses-permission android:name="android.permission.POST_NOTIFICATIONS"/>
|
Initialize FCM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class MainActivity : BaseActivity() {
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main)
... Firebase.messaging.isAutoInitEnabled = true FirebaseMessaging.getInstance().token.addOnCompleteListener(OnCompleteListener { task -> if (!task.isSuccessful) { return@OnCompleteListener } }) } }
|
Implement FCMService
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
| class FCMService : FirebaseMessagingService() {
override fun onMessageReceived(remoteMessage: RemoteMessage) {
remoteMessage.notification?.let { noti -> noti.title?.let { title -> sendNotification(title, noti.body ?: "") }
Log.d("TAG", "Message Notification Body: " + noti.title?:"title empty") } }
override fun onNewToken(token: String) { super.onNewToken(token) println(token) }
private fun sendNotification(title: String, body: String) { val notifID = System.currentTimeMillis().hashCode() val intent = Intent(this, NotificationListActivity::class.java) intent.addFlags(Intent.FLAG_ACTIVITY_REORDER_TO_FRONT) intent.putExtra("notificationId", notifID) val contentIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_ONE_SHOT)
val defaultSoundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION) val notificationBuilder = NotificationCompat.Builder(this, "default") .setSmallIcon(R.drawable.ic_launcher) .setContentTitle(title) .setContentText(body)
.setAutoCancel(true) .setContentIntent(contentIntent) .setTicker("PushNotificationTest APP") .setSound(defaultSoundUri)
val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { val channelID = "pushnotificationtest"; val channel = this.createNotificationChannel( notificationManager, channelID, channelID, NotificationManager.IMPORTANCE_HIGH )
channel.setShowBadge(true) notificationManager?.createNotificationChannel(channel) notificationBuilder.setChannelId(channelID) }
notificationManager.notify(notifID , notificationBuilder.build()) }
@TargetApi(26) private fun createNotificationChannel( notificationManager: NotificationManager, id: String, name: String, importance: Int ): NotificationChannel { return if (notificationManager.getNotificationChannel(id) != null) { notificationManager.getNotificationChannel(id) } else { val notificationChannel = NotificationChannel(id, name, importance) notificationChannel.enableVibration(true) notificationChannel.enableLights(true) notificationChannel } } }
|
Add FCMService to Manifest
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.maochun.pushnotificationtest"> ... <uses-permission android:name="android.permission.POST_NOTIFICATIONS"/> <application ...> ... <service android:name=".FCMService" android:exported="false"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT" /> </intent-filter> </service> </application>
|